C++ Module 01
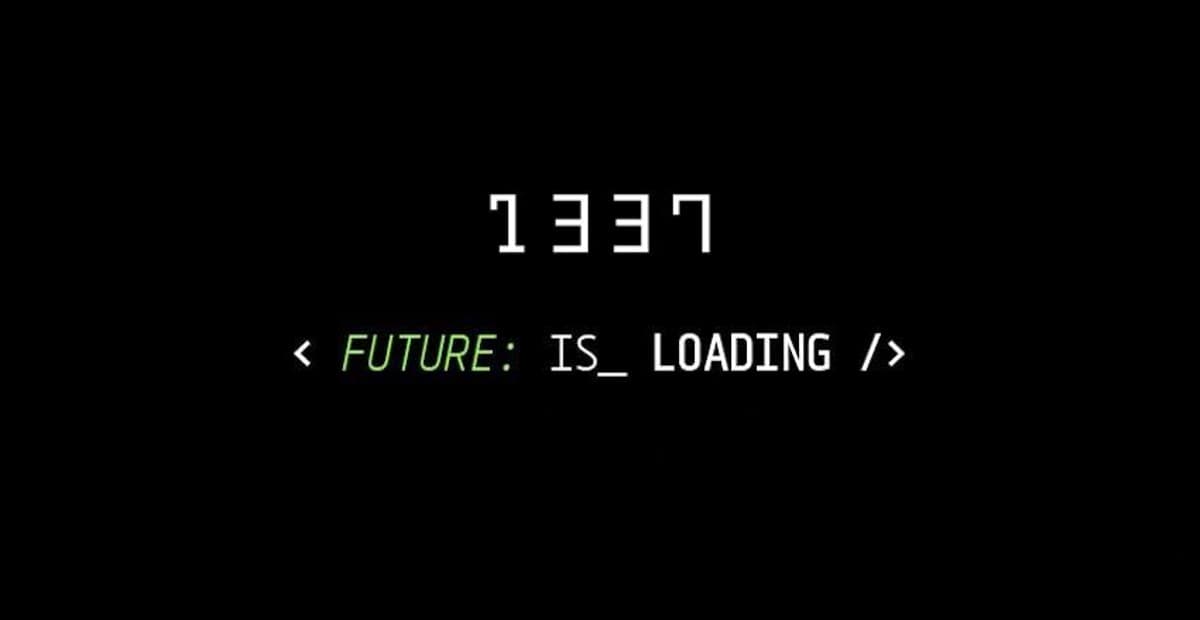
C++ Module 01: Exploring Memory Allocation and Pointers
Introduction
Module 01 of the C++ curriculum at 1337 coding school delves into memory allocation, pointers, references, and the switch statement. This module serves as a crucial step in introducing students to Object-Oriented Programming (OOP) within the C++ language.
General Rules
As with Module 00, adhering to general rules is essential. Compilation should be done with c++ and flags -Wall -Wextra -Werror, while code compliance with the C++98 standard is emphasized. The guidelines continue to encourage clean and readable code, providing flexibility in coding style.
Exercise 00: BraiiiiiiinnnzzzZ
Task:
Create a Zombie class with an announce function. Implement functions to create new zombies on the stack or heap, exploring the differences in memory allocation. Manage zombie destruction and debug using destructors.
Exercise 01: Moar brainz!
Task:
Implement a function zombieHorde that allocates a horde of Zombie objects in a single allocation. Test the function and ensure proper destruction of allocated memory.
Exercise 02: HI THIS IS BRAIN
Task:
Create a program that demonstrates memory addresses and values for a string variable using pointers and references.
Exercise 03: Unnecessary violence
Task:
Implement Weapon, HumanA, and HumanB classes. Explore when to use pointers and references for Weapon and understand the implications.
Exercise 04: Sed is for losers
Task:
Create a program that replaces occurrences of a specified string in a file with another string, without using C file manipulation functions.
Exercise 05: Harl 2.0
Task:
Design a Harl class with private member functions for different complaint levels. Implement a complain function that uses pointers to member functions. Test and ensure proper functionality.
Exercise 06: Harl filter
Task:
Implement a program that filters Harl's complaints based on log levels using the switch statement. Explore the switch statement for effective filtering.
Conclusion
Module 01 extends the foundational concepts introduced in Module 00. From memory allocation and pointers to implementing classes with specific functionalities, students gain a deeper understanding of C++ and its capabilities. The exploration of pointers to member functions and the switch statement further enriches their programming skills.
Remember, each exercise is an opportunity to strengthen your grasp of C++. Embrace the challenges, test your implementations thoroughly, and ensure compliance with the guidelines. Happy coding!