C++ Module 02
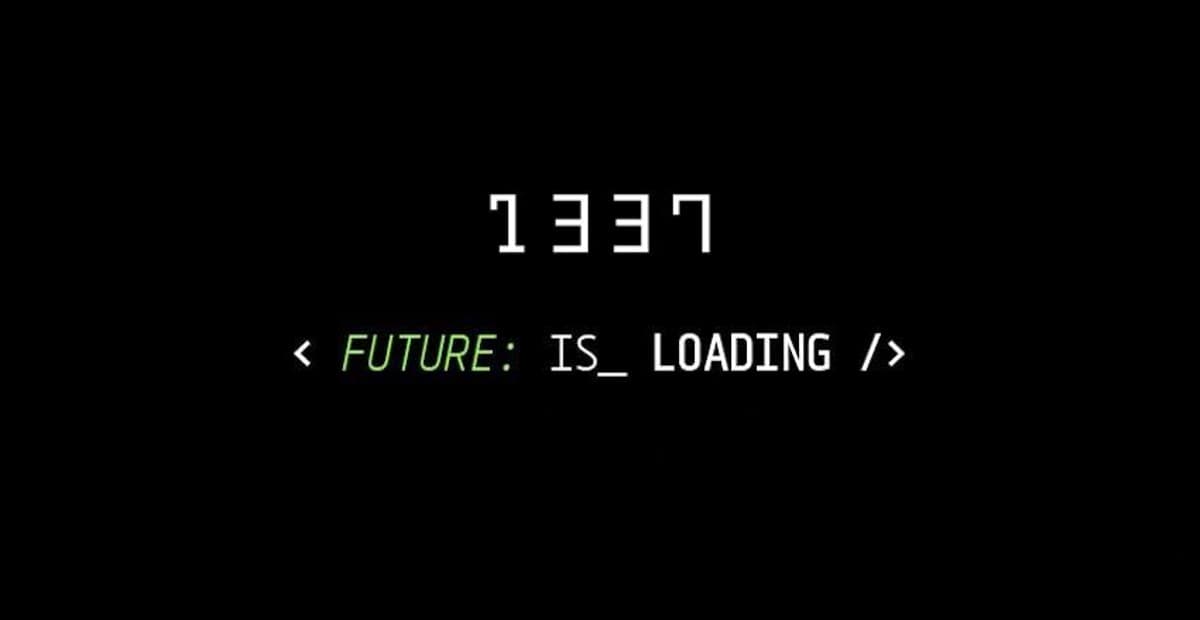
C++ Module 02: Polishing Fixed-Point Numbers and BSP Magic
Introduction
Module 02 of the C++ curriculum at 1337 coding school dives deeper into object-oriented programming, focusing on ad-hoc polymorphism, operator overloading, and the Orthodox Canonical class form. This module introduces the concept of fixed-point numbers and challenges students to implement a versatile Fixed class. Additionally, it explores binary space partitioning (BSP) with the creation of a Point class for geometric calculations.
General Rules Recap
The module retains the general rules from previous modules, emphasizing clean code, adherence to naming conventions, and proper use of C++ standards. Students are encouraged to read each module thoroughly before starting the exercises, ensuring a clear understanding of the requirements.
New Rules: Orthodox Canonical Form
A significant addition to the rules is the adoption of the Orthodox Canonical Form for class design. From this point forward, all classes must implement the four required member functions: default constructor, copy constructor, copy assignment operator, and destructor. The class code is split into header (.hpp/.h) and source (.cpp) files, promoting modular and organized code.
Exercise 00: My First Class in Orthodox Canonical Form
Task: Implement a Fixed class representing fixed-point numbers. The class includes private members for value and fractional bits, along with public constructors, conversion functions, and operators.
Exercise 01: Towards a More Useful Fixed-Point Number Class
Task: Enhance the Fixed class by adding constructors for integer and floating-point initialization. Implement conversion functions toFloat() and toInt(), and overload the insertion operator (<<) for easy output.
Exercise 02: Now We're Talking
Task: Further enrich the Fixed class by overloading comparison, arithmetic, and increment/decrement operators. Introduce static member functions min and max for comparing Fixed numbers.
Exercise 03: BSP
Task: Create a Point class in Orthodox Canonical Form to represent 2D points. Implement the bsp function that checks whether a point is inside a triangle using binary space partitioning.
Conclusion
Module 02 provides a comprehensive exploration of ad-hoc polymorphism, operator overloading, and class design principles. The implementation of the Fixed class demonstrates a practical application of fixed-point numbers, while the introduction of BSP adds a geometric dimension to the exercises. As students navigate the complexities of C++, they gain valuable insights into creating robust and efficient class structures.
Happy coding, and may your Fixed-point numbers and BSP algorithms shine brightly in the realm of C++!