C++ Module 03
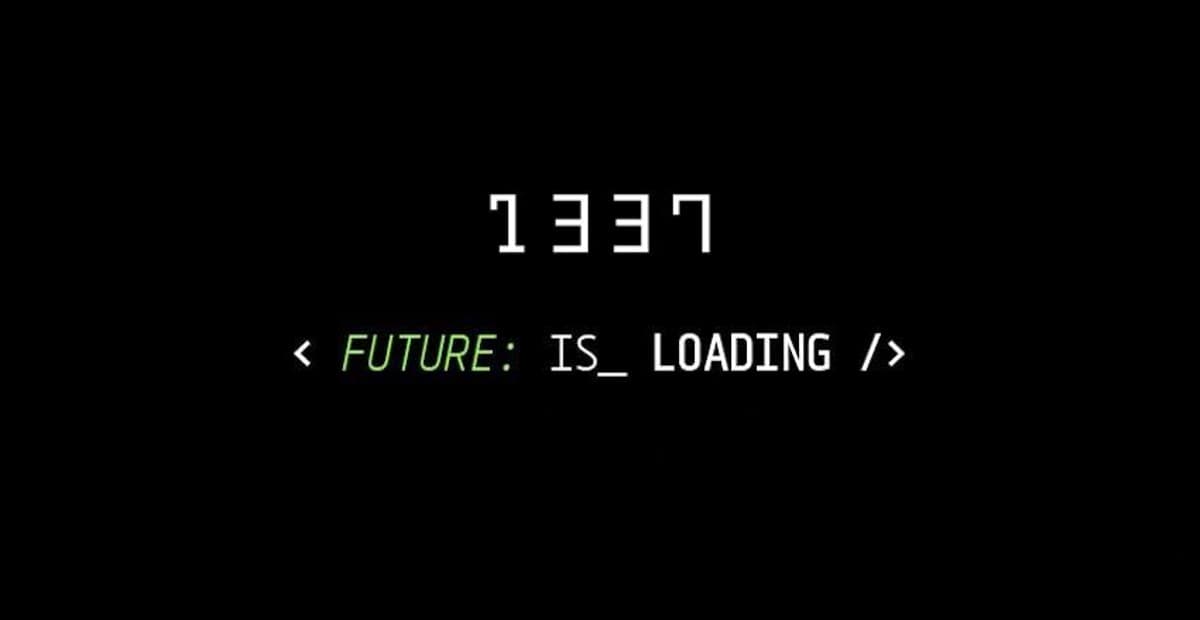
C++ Module 03: Embracing Inheritance and Robot Fusion
Introduction
Module 03 of the C++ curriculum at 1337 coding school explores the concept of inheritance in object-oriented programming. Students dive into creating a hierarchy of robot classes, each building upon the functionalities of its predecessors. The exercises involve designing and implementing classes that inherit attributes and behaviors, emphasizing proper construction/destruction chaining.
General Rules Recap
The general rules remain consistent, emphasizing clean code, adherence to naming conventions, and compliance with the C++98 standard. Students are encouraged to use the C++ standard library while adhering to design principles, such as the Orthodox Canonical Form.
Exercise 00: Aaaaand... OPEN!
Task: Implement the ClapTrap
class, representing a basic robot with attributes like name, hit points, energy points, and attack damage. Define member functions for attacking, taking damage, and being repaired. Ensure energy points and hit points constraints are enforced.
Exercise 01: Serena, my love!
Task: Create the ScavTrap
class, a derived robot inheriting from ClapTrap
. Customize the construction, destruction, and attack messages. Introduce a new member function, guardGate()
, exclusive to ScavTrap
. Demonstrate proper construction/destruction chaining.
Exercise 02: Repetitive work
Task: Design the FragTrap
class, another derived robot inheriting from ClapTrap
. Customize construction, destruction, and attack messages. Add a unique member function, highFivesGuys()
, and ensure proper construction/destruction chaining.
Exercise 03: Now it’s weird!
Task: Embark on the creation of DiamondTrap
, a robot that inherits from both FragTrap
and ScavTrap
. Overcome the challenge of dealing with multiple parent classes and handle attribute and member function conflicts. Introduce a unique member function, whoAmI()
, that displays both its name and its ClapTrap
name. Experiment with constructor chaining.
Conclusion
Module 03 offers a hands-on exploration of inheritance, enabling students to create a hierarchy of robot classes with distinct attributes and behaviors. The exercises progressively challenge students to design and implement more complex classes, showcasing the power and flexibility of inheritance in C++. As students navigate the intricacies of class hierarchies, they gain valuable insights into creating modular and extensible code.
Happy coding, and may your robotic creations conquer the realm of inheritance in C++!