Minishell
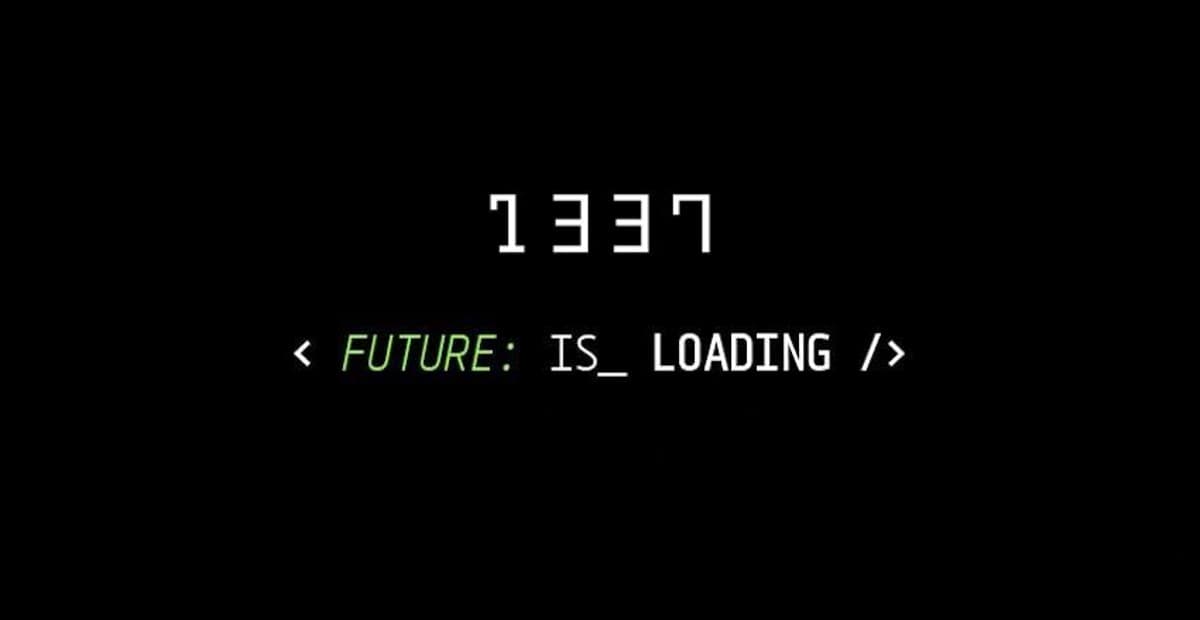
Minishell: A Journey into Shell Programming
Introduction
Welcome to the world of shell programming with Minishell! In this project, you'll embark on a journey to create your own simple shell, akin to the legendary bash. This project is not just about writing code; it's about understanding processes, file descriptors, and the essence of communication between humans and computers through commands.
Common Instructions
Before diving into the specifics of Minishell, let's revisit some common instructions applicable to all projects:
- Your implementation must be in C.
- Adhere to the Norm, including bonus files/functions in the norm check.
- Ensure functions don't quit unexpectedly; unexpected errors lead to non-functional projects.
- Free heap memory appropriately; no memory leaks are tolerated.
- Include a Makefile with essential rules:
$(NAME)
,all
,clean
,fclean
, andre
. - Separate bonus parts in
_bonus.{c/h}
if not specified otherwise. - If you use your
libft
, include its sources and Makefile in alibft
folder.
Mandatory Part: The Birth of Minishell
Program Name: minishell
Turn-in Files: Makefile, *.h, *.c
Arguments
External Functions:
- readline, rl_clear_history, rl_on_new_line, rl_replace_line, rl_redisplay, add_history, printf, malloc, free, write, access, open, read, close, fork, wait, waitpid, wait3, wait4, signal, sigaction, sigemptyset, sigaddset, kill, exit, getcwd, chdir, stat, lstat, fstat, unlink, execve, dup, dup2, pipe, opendir, readdir, closedir, strerror, perror, isatty, ttyname, ttyslot, ioctl, getenv, tcsetattr, tcgetattr, tgetent, tgetflag, tgetnum, tgetstr, tgoto, tputs Libft Authorized: Yes
Description
Build your own shell, and make it:
- Display a prompt when waiting for a new command.
- Have a working history.
- Search and launch the right executable based on the PATH variable or using a relative or absolute path.
- Use only one global variable.
- Not interpret unclosed quotes or special characters not required by the subject.
- Handle single quotes and double quotes correctly.
- Implement redirections:
<
,>
,<<
, and>>
. - Implement pipes (
|
) for command pipelines. - Handle environment variables (
$
followed by a sequence of characters). - Handle
$?
to expand to the exit status of the most recently executed foreground pipeline. - Handle
ctrl-C
,ctrl-D
, andctrl-\
as in bash. - Implement builtins:
echo
,cd
,pwd
,export
,unset
,env
,exit
.
Mandatory Part: The Core of Minishell
Your shell should be robust, handling various scenarios and functionalities as described in the project's instructions. Ensure the correct parsing and execution of commands, redirections, pipes, and builtins.
Bonus Part: Elevating Minishell
The bonus part allows you to enhance your Minishell further. Implement the following features if the mandatory part is perfect:
- Implement
&&
and||
with parenthesis for priorities. - Allow wildcards
*
to work for the current working directory.
Conclusion
Minishell is not just a project; it's an exploration of the intricate world of shell programming. Embrace the challenges, build a robust shell, and learn the art of command-line interaction. Happy coding, and may your Minishell shine brightly in the realm of shells!