Philosophers
Amine Beihaqi
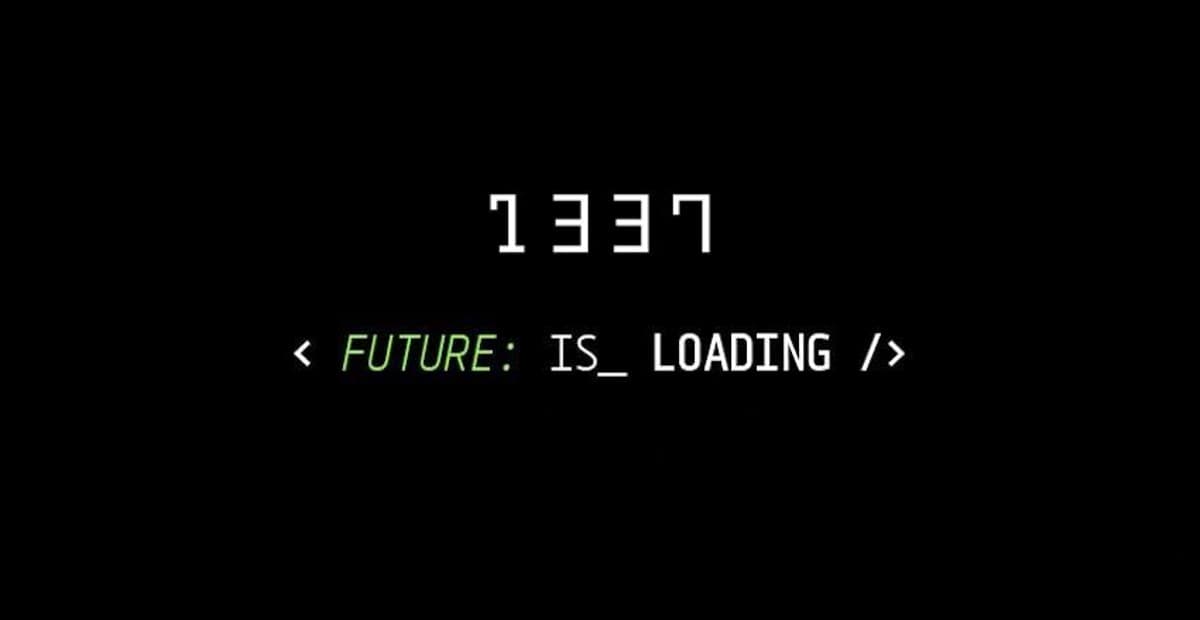
Amine Beihaqi
Philosophers: A Multithreaded Concurrency Challenge
Project Overview
The Philosophers project is an advanced systems programming challenge that explores concurrent programming, thread synchronization, and resource management through a metaphorical dining scenario. By implementing a sophisticated simulation of philosophers sharing limited resources, the project provides a deep dive into the complexities of parallel computing.
Technical Concept
The Philosophical Dilemma
The project models a classic concurrent programming problem: philosophers seated around a table, each requiring two forks to eat. This scenario serves as a compelling framework for exploring critical synchronization challenges:
- Resource contention
- Deadlock prevention
- Fair resource allocation
- Real-time process management
Implementation Approaches
Mandatory Solution: Threaded Implementation
- Utilizes POSIX threads (pthreads)
- Implements mutex-based fork management
- Handles up to 200 philosophers simultaneously
- Precise timing control with millisecond-level accuracy
Bonus Solution: Process-Based Approach
- Implements philosophers as separate processes
- Manages resources using semaphores
- Demonstrates inter-process communication
- Provides an alternative synchronization strategy
Key Technical Challenges
Synchronization Mechanics
- Preventing deadlocks
- Ensuring fair fork distribution
- Avoiding philosopher starvation
- Implementing precise state tracking
Performance Constraints
- Zero memory leaks
- Minimal computational overhead
- Real-time state change monitoring
- Death detection within 10 milliseconds
Technical Specifications
Programming Environment
- Language: C
- Synchronization Primitives:
- POSIX Threads
- Mutex locks
- Semaphores
- System-level process management
Skills Demonstrated
- Advanced concurrent programming
- Low-level system design
- Resource synchronization
- Performance optimization
- Complex algorithm implementation
Project Significance
Beyond a theoretical exercise, this project provides a practical exploration of:
- Concurrent system design principles
- Real-world synchronization challenges
- Efficient resource management strategies
GitHub Repository
Technical Skills Highlighted
- Multithreaded programming
- Process synchronization
- Low-level systems programming
- Algorithmic problem-solving
- Performance-critical design